Conditional rendering is a pivotal concept in ReactJS development, enabling developers to create dynamic user interfaces that adapt to changing conditions and user interactions. Understanding and effectively implementing conditional rendering techniques is essential for crafting intuitive and responsive applications. In this blog post, we’ll delve into the fundamentals of conditional rendering in ReactJS, explore various approaches, and provide insightful examples to illustrate its power.
Table of Contents
What is Conditional Rendering?
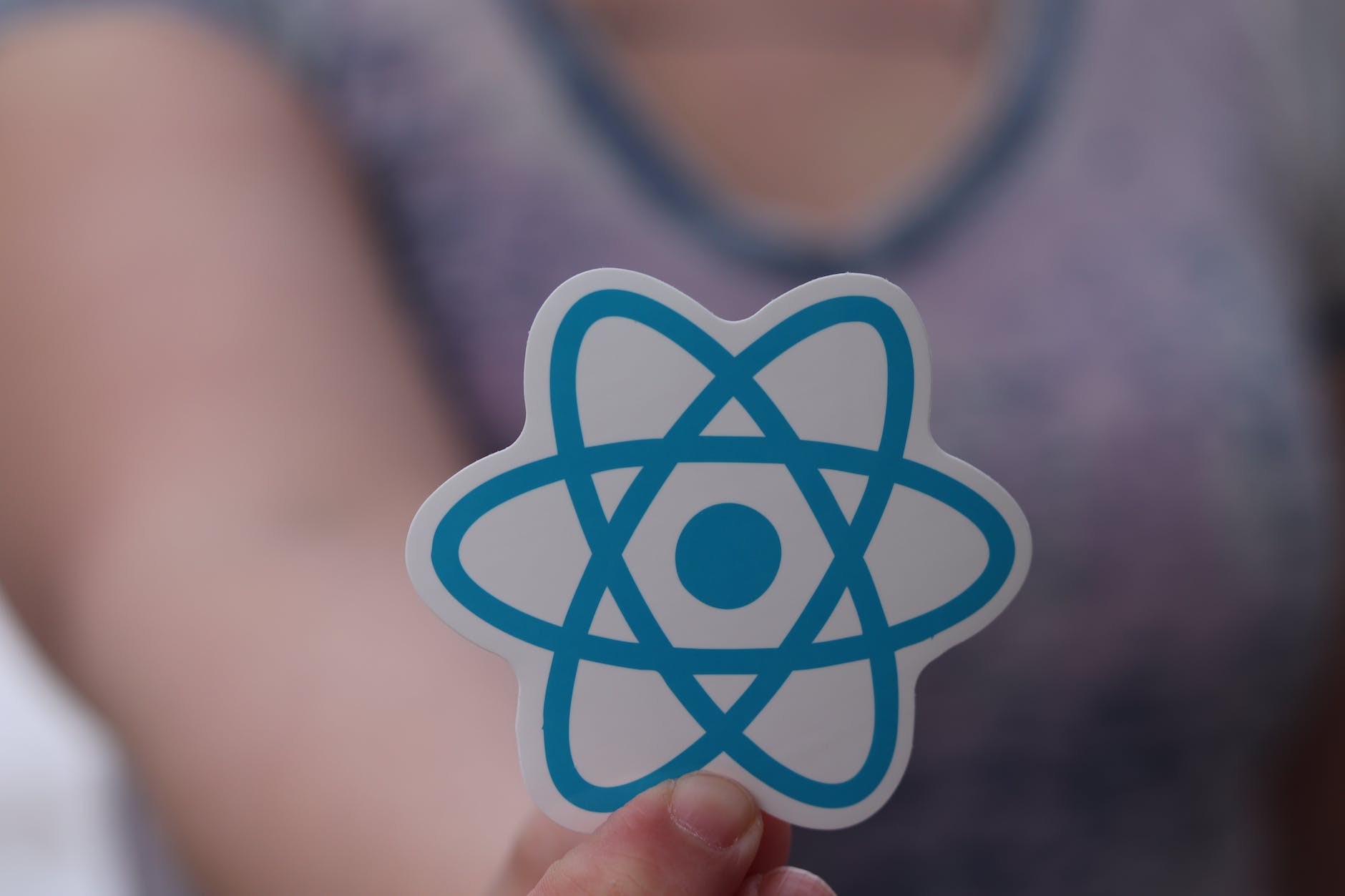
Conditional rendering in ReactJS involves selectively rendering components or UI elements based on certain conditions. This flexibility allows developers to display different content or user interfaces dynamically, depending on factors such as user input, data availability, or application state. By leveraging conditional rendering, React developers can create interactive and personalized experiences that cater to diverse user scenarios.
Conditional Rendering Techniques
ReactJS offers several techniques for implementing conditional rendering, each suited to different use cases. Some common approaches include:
- Using JavaScript Conditional Statements: Developers can employ standard JavaScript conditional statements such as
if
,else if
, andelse
within JSX to conditionally render components. This approach provides straightforward logic for displaying different UI elements based on specific conditions. - Ternary Operator: The ternary operator (
condition ? true : false
) offers a concise way to perform conditional rendering within JSX. It enables developers to express conditional logic in a compact and readable manner, making the code more concise and expressive. - Logical && Operator: Leveraging the logical AND (
&&
) operator allows for conditional rendering based on truthy values. When the condition evaluates totrue
, the subsequent component or element is rendered, providing a concise way to conditionally render content. - Using Inline Functions: Developers can define inline functions within JSX to encapsulate conditional rendering logic. This approach enables dynamic computation of component rendering based on complex conditions or state variables.
Example: Conditional Rendering in Action
Let’s consider a practical example to illustrate conditional rendering in ReactJS. Suppose we’re building a user authentication system where we want to display a welcome message if the user is logged in and a login prompt if they’re not. Here’s how we can implement this scenario:
import React from 'react';
function App() {
const isLoggedIn = true; // Assuming user is logged in
return (
<div>
{isLoggedIn ? (
<WelcomeMessage />
) : (
<LoginPrompt />
)}
</div>
);
}
function WelcomeMessage() {
return <h1>Welcome back!</h1>;
}
function LoginPrompt() {
return <p>Please log in to continue.</p>;
}
export default App;
In this example, the isLoggedIn
variable determines whether to render the <WelcomeMessage />
component or the <LoginPrompt />
component based on the user’s authentication status.
Conclusion
Conditional rendering is a fundamental aspect of ReactJS development, enabling developers to create dynamic and interactive user interfaces. By mastering conditional rendering techniques, developers can build applications that adapt seamlessly to user interactions and changing conditions. Whether using JavaScript conditional statements, ternary operators, logical operators, or inline functions, understanding these approaches empowers developers to craft intuitive and responsive React applications.
Incorporating conditional rendering into your React projects opens up endless possibilities for creating engaging user experiences and enhancing application functionality. Embrace the power of conditional rendering and unlock the full potential of ReactJS development.