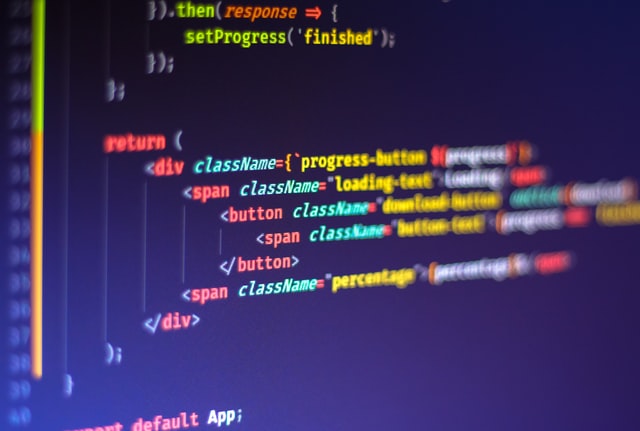
In this article I am going to show you how to create a very basic and simple web app using HTML, CSS and JAVASCRIPT where you can receive JSON data from an API and display them on the front end.
I am assuming you already have a basic knowledge of HTML, CSS and JAVASCRIPT. If you already don’t know what HTML, CSS and JS is, then I would recommend you to just go through some tutorials online ( Search google / youtube). Here is one article that I have made recently. Once you have some basic understanding of HTML, CSS and JAVASCRIPT then you can come back here.
WHAT IS AN API :
API stands for Application Programming Interface which helps two applications to talk to each other. A call to the API needs to be initiated from the client side. In our case we are building that client using HTML (index.html), CSS ( style.css) and Javascript ( script.js ).
In the script.js we initiate the API call using JavaScript fetch() method. Once we receive the response in the form of JSON ( JavaScript Object Notation as (key, value) pair then we process it and store it in a local variable. After that we display the response data in table format with the help of HTML and CSS.
That’s all !!
For the record I will be creating this tutorial for Windows users only.
Okay, So i will be creating this simple app on my most favourite editor “Visual Studio Code “.
So please download and install VSCODE from here.
If you have any other choice of editors, you are most welcome. If you don’t know what an editor is, it is an application that helps to write and manage our code. There are plenty of nice editors out there e.g Bracket, Sublime text, Atom , Web storm, Netbeans, Eclipse etc. I personally like VSCODE a lot as it is fast and provides a gazillions of plugins to install in a blink of your eye based on your need.
STEPS :
————
#OPEN YOUR COMMAND PROMPT ( CMD )
Table of Contents
To Create a directory / folder in command prompt
Type
mkdir “directoryname” ( e.g. mkdir testWebApi)
Then type
cd “directoryname” (e.g. cd testWebApi )
Next Type
code .
This will directly open the folder in VSCODE
#DUMMY API
I will be using a dummy ( freely available online ) API. In this tutorial I won’t teach you how to create an API.
I will be using this API
You can test this API using some tools like POSTMAN / INSOMNIA or you can just directly copy and paste it in your web browser ( for me it’s chrome ) and see what’s the response coming. This is what it looks like in the screenshot below when you paste the link in your browser URL and hit the “enter” key.
The API gives us a JSON response in the form of ( key, value) pair.
#CREATE FILES IN VSCODE
Create three files in VSCODE
INDEX.HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Input Form</title>
<link rel="stylesheet" href="style.css">
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
</head>
<body>
<table id="employees"></table>
<script src="script.js"></script>
</body>
</html>
style.css
table th {
border: 1px solid;
background-color: cyan;
}
table td {
border: 1px dotted;
text-align: center;
color: #fff;
padding: 10px;
}
table td:nth-child(1){
background-color: orange;
}
table td:nth-child(2){
background-color: green;
}
table td:nth-child(3){
background-color: black;
}
table td:nth-child(4){
background-color: red;
}
table {
margin-left: auto;
margin-right: auto;
margin-top: 100px;
}
SCRIPT.JS
const api_url = "https://jsonplaceholder.typicode.com/users";
async function getApiData(url) {
const response = await fetch(url);
var data = await response.json();
console.log(data);
show(data);
}
getApiData(api_url)
function show(data) {
let tab = `<tr>
<th>ID</th>
<th>NAME</th>
<th>USER NAME</th>
<th>EMAIL</th>
</tr>`;
for (let r of data) {
tab += `<tr>
<td>${r.id} </td>
<td>${r.name}</td>
<td>${r.username}</td>
<td>${r.email}</td>
</tr>`;
}
document.getElementById("employees").innerHTML = tab;
}
To Run this app you can directly go to the folder testWebApI and double click on index.html
or you can install a plugin in VSCODE ( my favourite ) called “live server”. Once you have installed the plugin you can right click on the HTML code ( in index.html) and click “Open with live server”.
OUTPUT :
—————-
We get the following output on Browser…
Hope this article helps you to understand what an API is and how to call and retrieve JSON data from an API and to display it on the browser.
References :
- https://www.geeksforgeeks.org/
- https://www.w3schools.com/
Giving is not just about making donations, it’s about making a difference !! Content Shark needs your little help to keep this community growing !!
Reference :