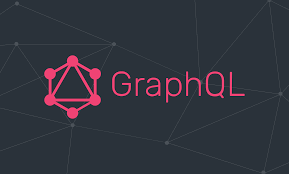
GraphQL has revolutionized how we fetch data in web development. As a query language and server-side runtime, GraphQL is designed to optimize data fetching for client applications. It allows clients to specify the exact data they need, eliminating the inefficiencies of traditional REST APIs. This blog will delve into the benefits of GraphQL, compare it to REST, and explore its core concepts and components, making it clear why this technology is essential for modern application development.
Table of Contents
The Basics of GraphQL
GraphQL, developed by Facebook in 2012 and released as an open-source project in 2015, is both a query language for APIs and a runtime for executing those queries. Unlike traditional REST APIs, which are tied to specific databases or storage engines, GraphQL is backed by your existing code and data. This flexibility ensures that when a client requests data from a server, only the exact data needed is retrieved, avoiding over-fetching or under-fetching information.
The Problem with Traditional REST APIs
Over-Fetching
In traditional RESTful APIs, data is often fetched through endpoints that return complete resources or collections of resources. Consider a scenario where you need to display the salary of an employee based on their employee ID. Using a REST API, you might receive a response like this:
[
{
"empId": 1001,
"empName": "John",
"empAddress": {
"city": "XYZ",
"pincode": "123456",
"country": "USA",
"street": "El Camino Rd",
"aptNo": "127 Niles Canyon Road"
},
"empMaritalStatus": "Married",
"empSalary": "$2000"
}
]
In this example, to obtain the empSalary, the entire employee record is fetched, including unnecessary details like empName, empAddress, and empMaritalStatus. This means retrieving more data than necessary, increasing the load on the server and the client.
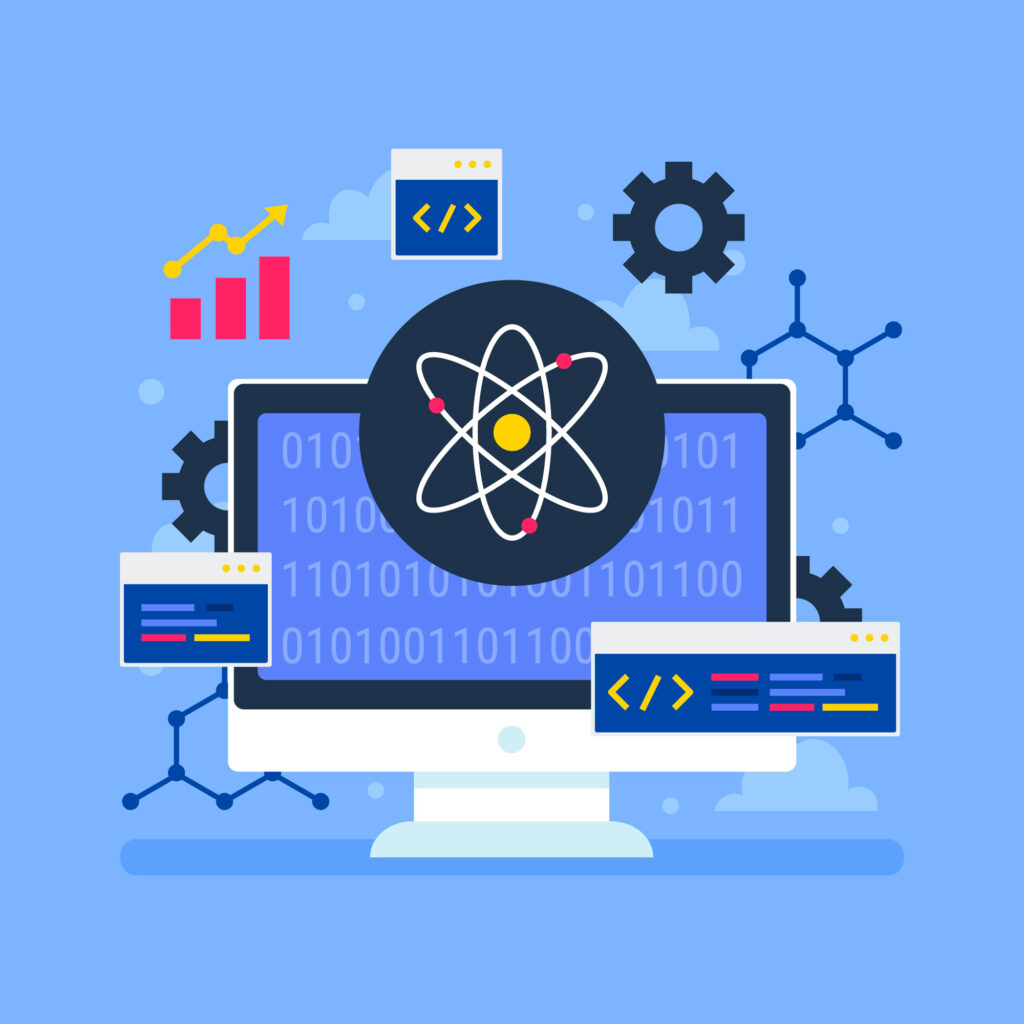
Under-Fetching
Under-fetching occurs when the server does not provide enough data to fulfill a specific task. This happens when the server provides only basic information, lacking details needed for decision-making. For example, an e-commerce site might display products with basic information but lack details such as price, description, or availability, preventing users from making informed decisions.
How GraphQL Solves These Problems
GraphQL addresses these inefficiencies by allowing clients to specify exactly what data they need and nothing more. Acting as an intermediary between the client and the data sources, GraphQL filters the data based on the query specified by the client. This ensures that only the required data is fetched and transmitted, significantly reducing server load and improving application performance.
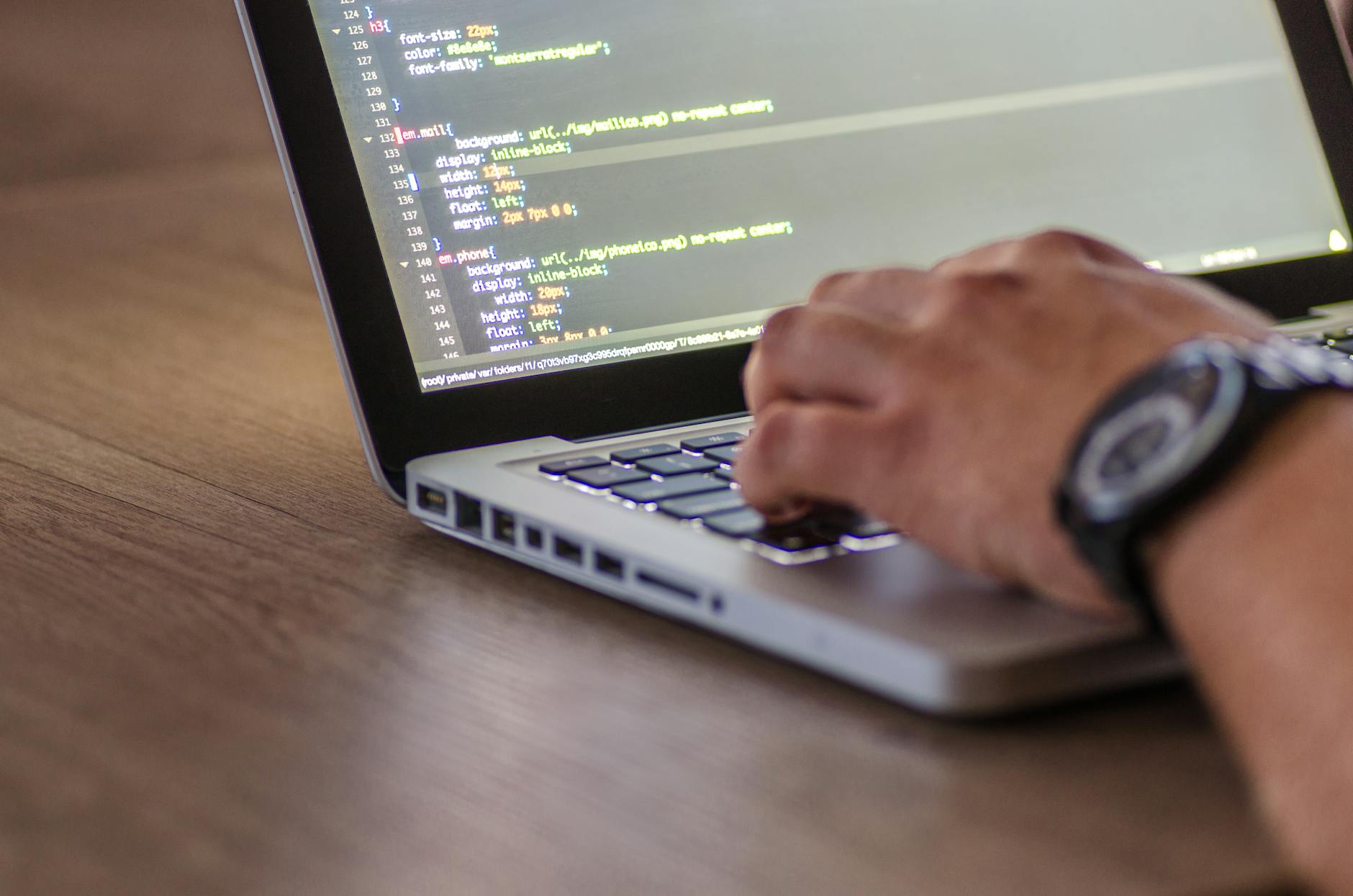
Here’s how you could query just the salary of an employee using GraphQL:
query {
employee(empId: 1001) {
empSalary
}
}
The server responds with precisely what is requested:
{
"data": {
"employee": {
"empSalary": "$2000"
}
}
}
Benefits of Using GraphQL
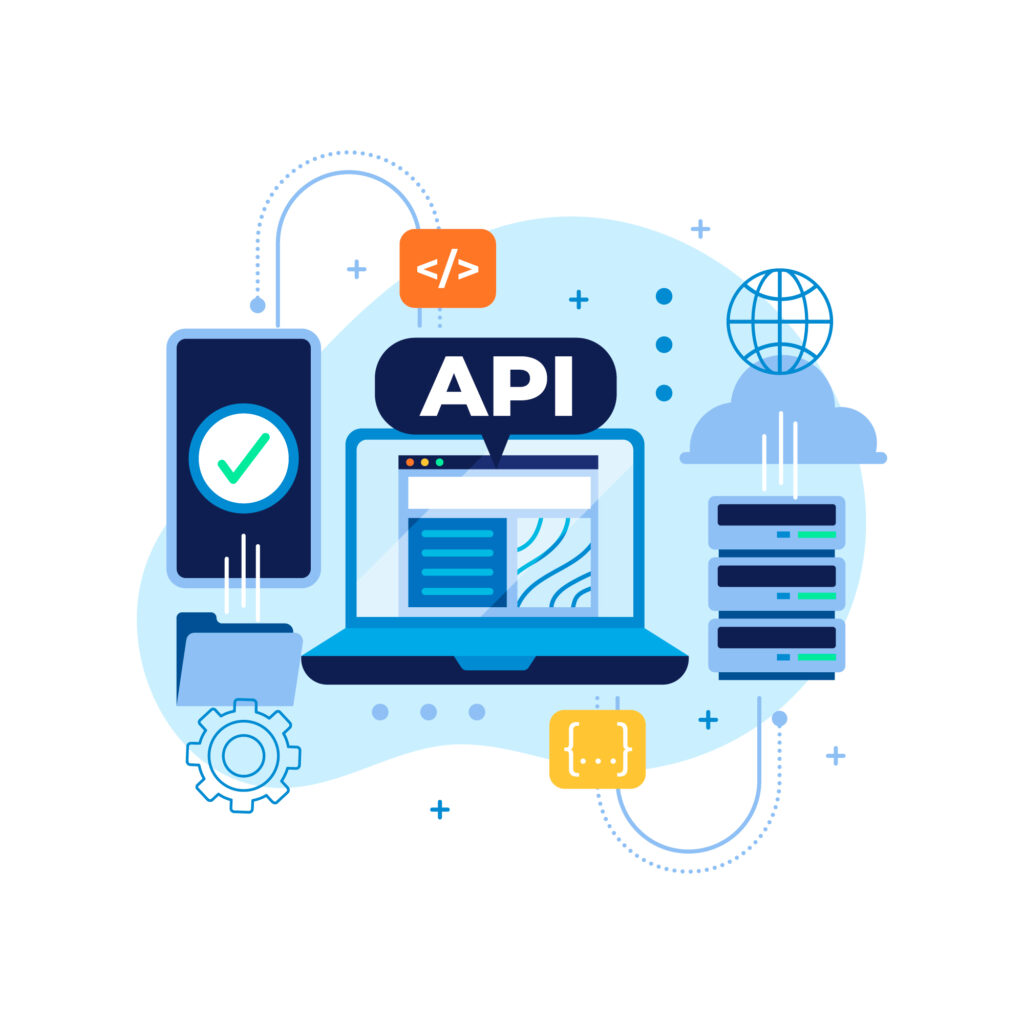
Precise Data Fetching
- Exact Fields: GraphQL allows clients to request only the specific fields they need, avoiding the overhead of fetching entire records or unnecessary fields.
- Nested Queries: It supports querying nested related data in a single request, which is particularly useful for complex data models.
Improved Performance
- Reduced Data Transfer: By fetching only the necessary data, GraphQL minimizes the amount of data sent over the network.
- Batching and Caching: GraphQL servers can batch multiple requests into a single query and cache results, improving efficiency and reducing load times.
Flexibility and Scalability
- Single Endpoint: GraphQL APIs are accessed through a single endpoint, simplifying the API structure and making it easier to evolve over time without versioning.
- Dynamic Queries: Clients can query different shapes and forms of data without requiring changes to the backend or additional endpoints.
Enhanced Developer Experience
- Strong Typing: GraphQL schemas are strongly typed, providing clear documentation and helping with debugging and tooling support.
- Introspection and Documentation: GraphQL’s introspection capabilities allow for automatic documentation generation and powerful development tools.
Real-Time Capabilities
- Subscriptions: GraphQL supports real-time data updates through subscriptions, allowing clients to receive updates whenever the data changes.
Drawbacks of REST APIs
- Over-fetching: Retrieving more data than necessary.
- Under-fetching: Retrieving insufficient data for specific tasks.
- Multiple API Calls: Often, multiple REST API calls are needed to get the required data.
GraphQL Features
In GraphQL, resolvers handle different types of operations, including queries, mutations, and subscriptions:
- Queries: Used to read or fetch data from the server, similar to GET requests in RESTful APIs.
- Mutations: Used to write or modify data on the server, similar to POST, PUT, PATCH, or DELETE requests in RESTful APIs.
- Subscriptions: Used to receive real-time updates from the server, similar to WebSocket connections.
Language Agnostic
GraphQL can extract data from any working API regardless of the language in which the APIs are built, as long as the APIs are accessible via HTTP. This means you can create a GraphQL server that interacts with APIs built using different technologies, such as Spring Boot (Java) and Node.js.
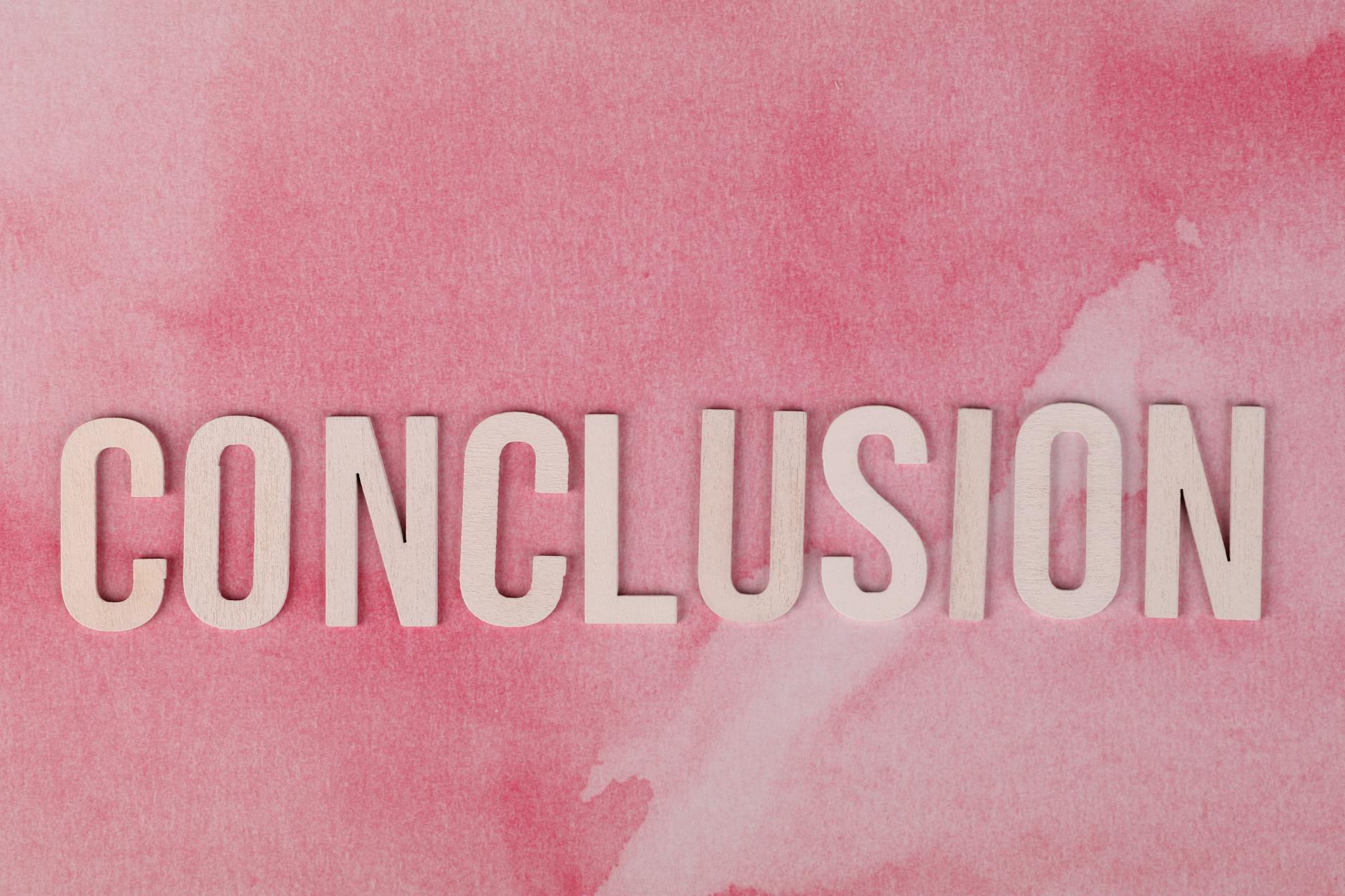
Conclusion
GraphQL offers a powerful alternative to traditional REST APIs by enabling precise and efficient data fetching. Its ability to request exactly what is needed, combined with features that enhance performance and developer productivity, makes it an excellent choice for modern application development. By reducing the load on servers and minimizing data transfer, GraphQL optimizes both the frontend and backend, providing a smoother and more efficient user experience.
What is ApolloServer ?
Apollo Server
Apollo Server is an open-source, community-maintained GraphQL server that can be used to build a GraphQL API quickly and efficiently. It provides tools and features that make it easy to define the schema, manage resolvers, and integrate with various data sources.
Key Features of Apollo Server
- Schema Definition: Define your GraphQL schema using the Schema Definition Language (SDL).
- Resolvers: Write resolver functions to fetch and manipulate data based on the defined schema.
- Integration: Seamlessly integrate with various data sources, including databases, REST APIs, and third-party services.
- Tooling: Enhance development experience with Apollo Studio for schema management, performance monitoring, and error tracking.
- Community and Ecosystem: Part of the larger Apollo ecosystem, which includes client libraries, development tools, and a strong community.
Apollo’s Ecosystem
- Apollo Server: Core GraphQL server for defining schema and resolvers.
- Apollo Studio: Cloud-based platform for managing, monitoring, and optimizing GraphQL APIs.
- Apollo Explorer: Interactive tool for running and debugging GraphQL operations.
- Apollo Sandbox: Interactive playground for testing and debugging your GraphQL API, accessible at the /graphql endpoint.
- Apollo Client: State management library for JavaScript applications using GraphQL.
- Apollo Federation: Technique for splitting a GraphQL schema into multiple subgraphs.
- Apollo Gateway: Aggregates multiple schemas into a single unified schema for clients.
- Apollo Tracing: Provides performance insights and metrics for GraphQL operations.
- Apollo Server Plugins: Extend and customize Apollo Server behavior.
Project 1: Creating a Simple GraphQL API using Apollo Server and GraphQL with Node.js
To create a simple GraphQL project using Node.js, we need to install two packages:
npm i apollo-server graphql
npm pkg set type="module" (This will help us to use the ES6 module system)
Create an index.js
file:
touch index.js
Before writing code in index.js
, we need to know about GraphQL data types:
GraphQL Data Types
- Scalar Types: Int, Float, String, Boolean, ID.
- Custom Scalars: Defined by the user (e.g., Date).
- Object Types: Structured types with fields.
- Enum Types: Predefined set of values.
- Input Object Types: Used for complex input arguments.
- Lists: Arrays of a specific type.
- Non-Null: Fields that cannot be null.
- Interfaces: Abstract types with common fields.
- Unions: Types that can be one of several types.
index.js Example
import { ApolloServer, gql } from 'apollo-server';
const typeDefs = gql`
type Book {
title: String
author: String
}
type Query {
books: [Book]
}
`;
const resolvers = {
Query: {
books: () => books,
},
};
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`🚀 Server ready at ${url}`);
});
const books = [
{
title: 'The Great Gatsby',
author: 'F. Scott Fitzgerald',
},
{
title: 'Wuthering Heights',
author: 'Emily Brontë',
},
];
Using #graphql
and gql
In Apollo Server and GraphQL development, you might encounter #graphql
and gql
. Both are related to defining GraphQL schemas and queries in a JavaScript or TypeScript environment.
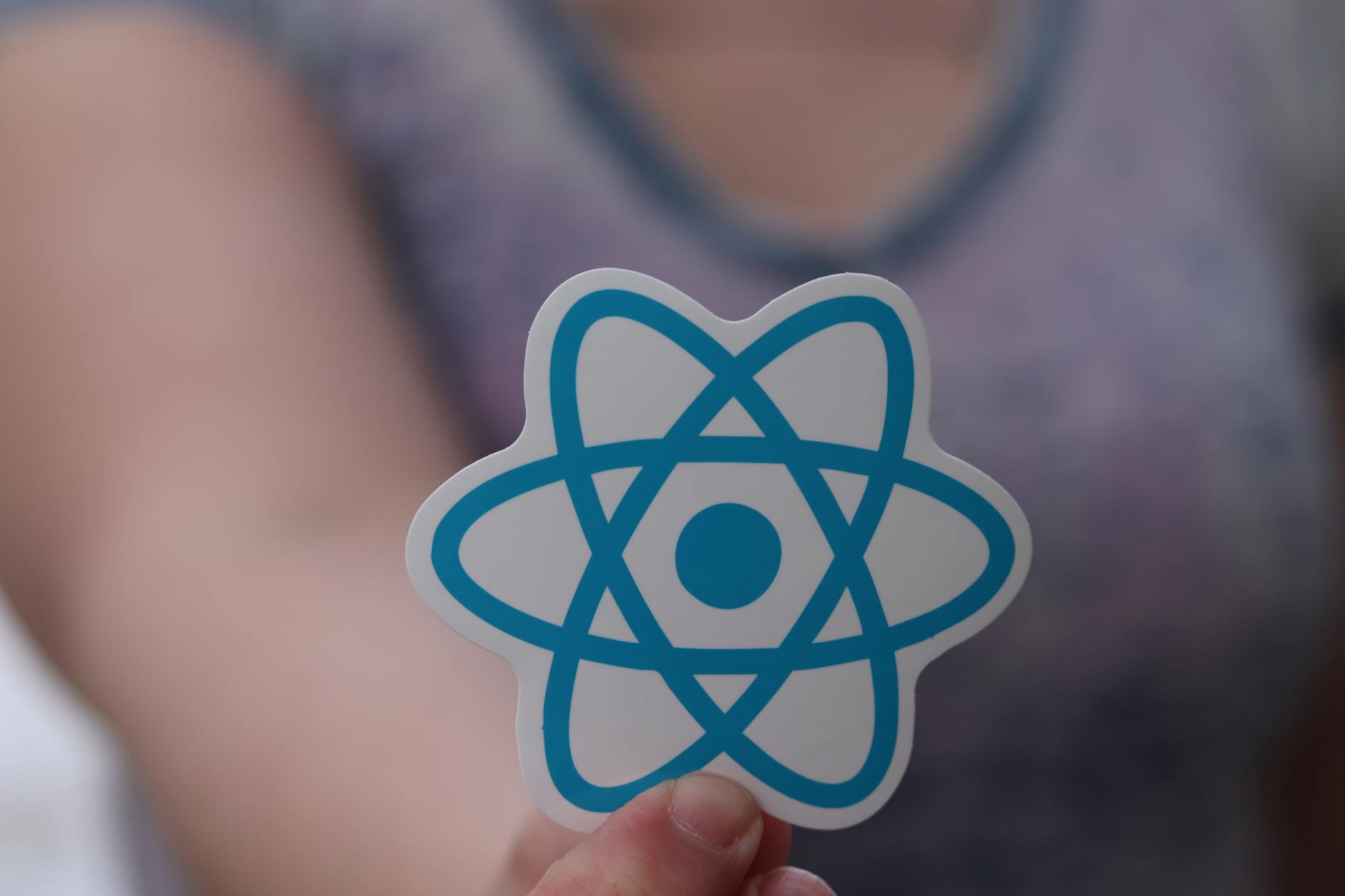
#graphql
#graphql
is a syntax highlighting comment used to indicate GraphQL code within a template literal. It doesn’t have a functional impact but can improve readability and developer experience when writing GraphQL schema definitions or queries in a JavaScript file.
gql
In Apollo Server, gql
is often used for parsing GraphQL schema strings, but it’s not strictly necessary if you’re defining the schema in a format that Apollo Server can understand directly. Using gql
provides benefits like syntax highlighting, linting, and parsing the schema into AST.
Conclusion
GraphQL and Apollo Server provide powerful tools for modern API development. By leveraging the precise data fetching capabilities of GraphQL and the robust ecosystem of Apollo, developers can build efficient, scalable, and flexible APIs. Whether you’re new to GraphQL or looking to deepen your understanding, these technologies offer a compelling approach to modern web development.