Table of Contents
What is Formik?
Formik is a popular open-source library for building and processing form data in React applications. It simplifies three major challenges developers face while working with HTML forms:
- Accessing the proper input values while the form is being submitted at a specific form state.
- Validating values entered against each specific form element and generating error messages if any at that very moment.
- Handling the submission of the form.
Formik also integrates seamlessly with Yup, a schema-based validation library, providing robust form validation capabilities.
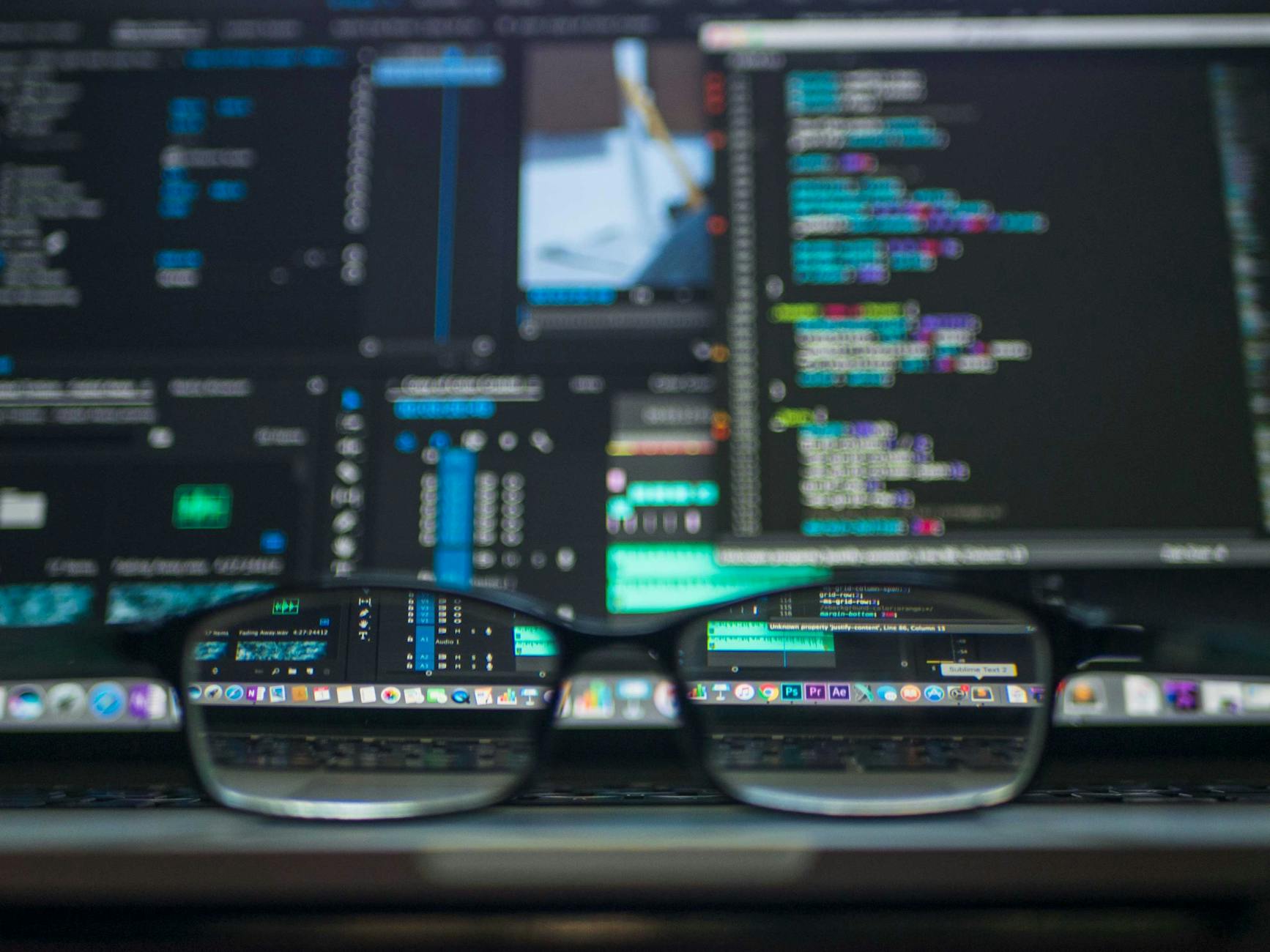
Installation
You can install Formik using npm or yarn:
npm i formik --save
or
yarn add formik
Basic Form Handling Without Formik
Here’s how you can handle a basic form using React’s state management:
import React, { useState } from 'react';
function BasicForm() {
const [inputValue, setInputValue] = useState('');
const handleChange = (event) => {
setInputValue(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
console.log(inputValue);
};
return (
<form onSubmit={handleSubmit}>
<label>
Input:
<input type="text" value={inputValue} onChange={handleChange} />
</label>
<button type="submit">Submit</button>
</form>
);
}
export default BasicForm;
While the above code is functional, it can become cumbersome to manage forms with multiple inputs and complex validation logic. Formik simplifies these tasks.
Form Handling Using Formik
Formik provides two main ways to handle forms:
- Using
<Formik>
,<Form>
,<Field>
, and<ErrorMessage>
components. - Using the
useFormik
hook.
Approach 1: Using Formik Components
import React from 'react';
import { Formik, Form, Field, ErrorMessage } from 'formik';
function FormikComponentForm() {
return (
<Formik
initialValues={{ email: '', password: '' }}
onSubmit={values => {
console.log(values);
}}
>
{({ handleSubmit }) => (
<Form onSubmit={handleSubmit}>
<label>Email:</label>
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" />
<label>Password:</label>
<Field type="password" name="password" />
<ErrorMessage name="password" component="div" />
<button type="submit">Submit</button>
</Form>
)}
</Formik>
);
}
export default FormikComponentForm;
Example 2: With Validation
import React from 'react';
import { Formik, Form, Field, ErrorMessage } from 'formik';
export default function TestFormik() {
return (
<div>
<Formik
initialValues={{ email: '', password: '' }}
validate={values => {
const errors = {};
const emailRegExPattern = /^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}$/i;
if (!values.email) {
errors.email = 'Email Required';
} else if (!emailRegExPattern.test(values.email)) {
errors.email = 'Invalid email address';
}
if (!values.password) {
errors.password = 'Password Required';
}
return errors;
}}
onSubmit={(values, { setSubmitting }) => {
setTimeout(() => {
alert(JSON.stringify(values, null, 2));
setSubmitting(false);
}, 400);
}}
>
{({ isSubmitting }) => (
<Form>
<Field type="email" name="email" placeholder="Email" />
<ErrorMessage name="email" component="div" />
<Field type="password" name="password" placeholder="Password" />
<ErrorMessage name="password" component="div" style={{color:'red'}} />
<button type="submit" disabled={isSubmitting}>
Submit
</button>
</Form>
)}
</Formik>
</div>
);
}
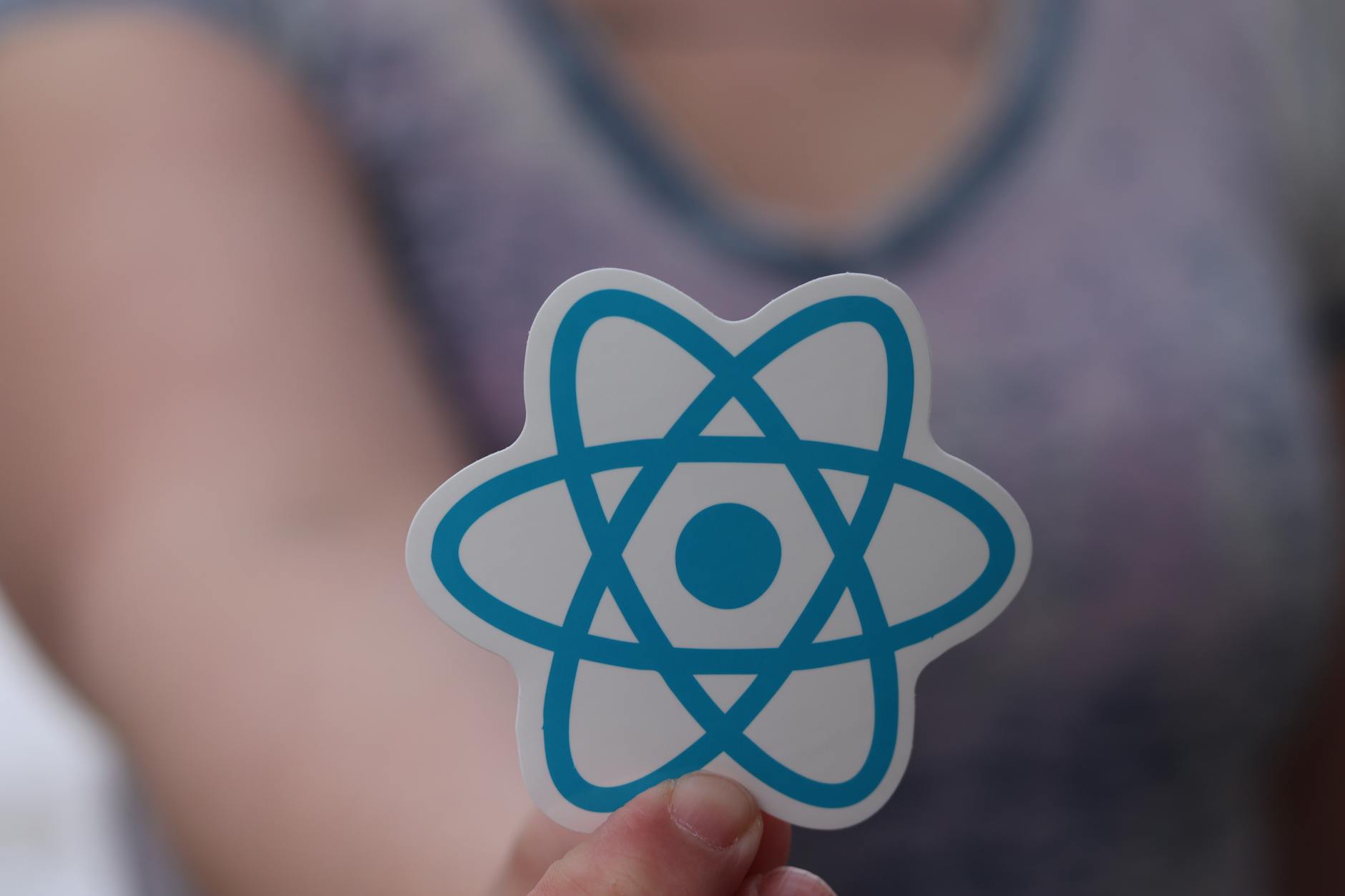
Approach 2: Using useFormik
Hook
import React from 'react';
import { useFormik } from 'formik';
function SimpleFormikForm() {
const formik = useFormik({
initialValues: {
email: '',
password: ''
},
onSubmit: values => {
console.log(values);
}
});
return (
<form onSubmit={formik.handleSubmit}>
<label>Email:</label>
<input type="email" name="email" onChange={formik.handleChange} value={formik.values.email} />
<label>Password:</label>
<input type="password" name="password" onChange={formik.handleChange} value={formik.values.password} />
<button type="submit">Submit</button>
</form>
);
}
export default SimpleFormikForm;
Validation with Yup
Yup provides schema-based validation for form values. Integrating Yup with Formik allows you to handle form validation easily.
Installation
You can install Yup using npm or yarn:
npm i yup
or
yarn add yup
import React from 'react';
import { Formik, Form, Field, ErrorMessage } from 'formik';
import * as Yup from 'yup';
const validationSchema = Yup.object({
email: Yup.string().email('Invalid email format').required('Required'),
password: Yup.string().min(8, 'Password must be at least 8 characters').required('Required')
});
export default function YupFormik() {
return (
<Formik
initialValues={{ email: '', password: '' }}
validationSchema={validationSchema}
onSubmit={values => {
console.log('Form data', values);
}}
>
{({ handleSubmit }) => (
<Form onSubmit={handleSubmit}>
<label>Email:</label>
<Field type="email" name="email" /><br />
<ErrorMessage name="email" component="div" />
<label>Password:</label>
<Field type="password" name="password" /><br />
<ErrorMessage name="password" component="div" />
<button type="submit">Submit</button>
</Form>
)}
</Formik>
);
}
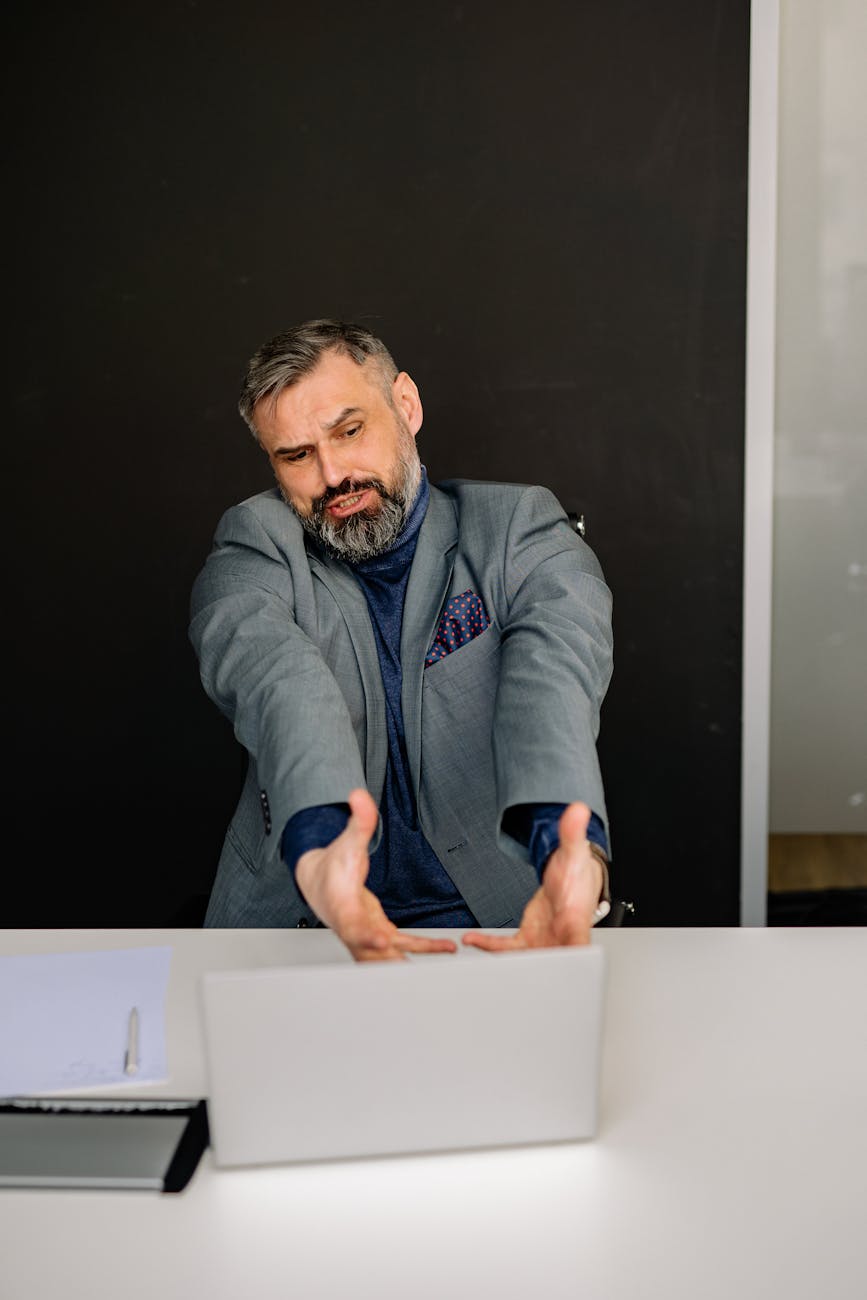