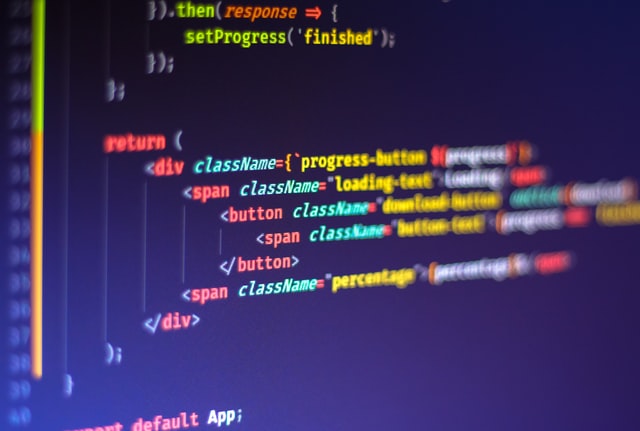
There are three different ways to declare variables in JavaScript. lets discuss the difference between them.
VAR :
var a = 100;
var a = 100;
var a = 101;
a = 200;
console.log(a)
———
Output : 200
—————————————–
LET
let a = 100;
a = 101
console.log(a)
Output : 101
——————————————-
But for the following case it will give an error,
let a = 100;
let a = 100;
let a = 101;
console.log(a);
SyntaxError: Identifier ‘a’ has already been declared
Now lets consider the following piece of code.
——————————————
Table of Contents
Using VAR
function varTest() {
var x = 1;
{
var x = 2; // same variable!
console.log(x); // 2
}
console.log(x); // 2
}
OUTPUT :
2
2
USING LET
function letTest() {
let x = 1;
{
let x = 2; // different variable
console.log(x); // 2
}
console.log(x); // 1
}
OUTPUT :
2
1
————————————————————————————–
CONST
const a = 100;
const a = 100;
const a = 101;
a = 101;
———-
In any of the above cases it will show some error
Assignment to constant variable
- So var works in a global scope.
- Let works in a local scope. Outside of the scope its value automatically gets de-assigned.
- Const is something similar to let but , in any case we can not the value. It’s more stricter than let. Also for const we have to initialize the moment we declare it.
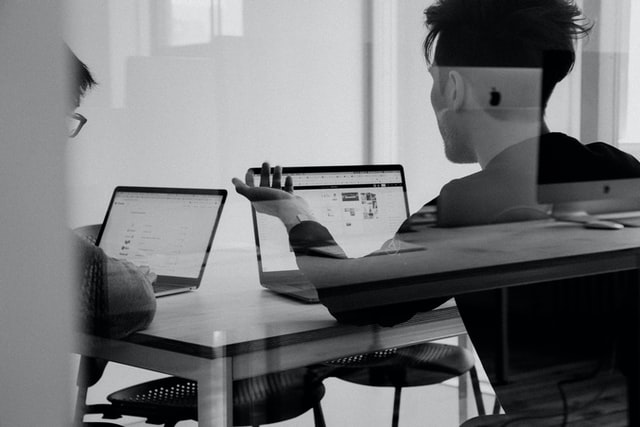
If you run this following code, it will throw an error if we don’t initialize the const variable
const a;
a = 100;
You will get an error.
There is another significant difference between var and let.
Because var works in the global scope so if any variable is being accessed before initializing it, it will be hoisted and will be assigned an “undefined” value.
console.log(b);
var b;
If you run the above code , you will get an “undefined” value.
But in-case of let it won’t be accessible and it will give an error.
console.log(b);
let b;
Cannot access ‘b’ before initialization
I could not refrain from commenting. Exceptionally well written!
thanks very much !!
Awesome article.
thanks very much !!
Usually I do not learn article on blogs, however I would like
to say that this write-up very compelled me to try and do so!
Your writing taste has been surprised me. Thanks,
quite great article.
thanks very much !!