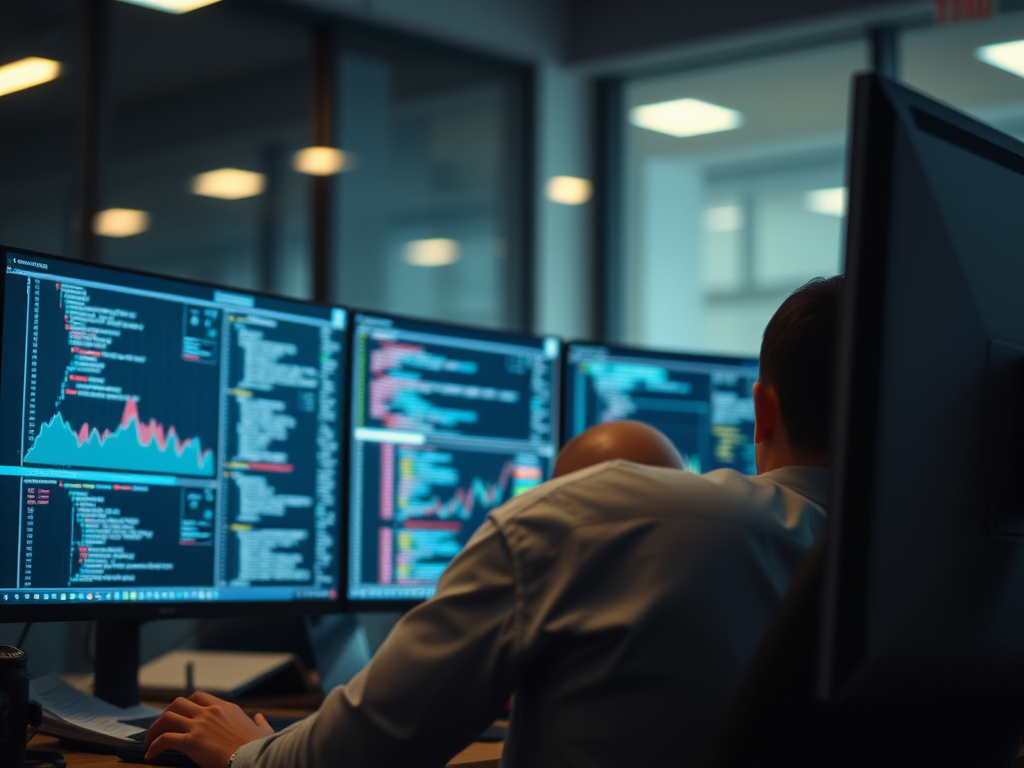
Table of Contents
Importance of DSA
Data structures are essential for the efficient storage of and access to data. While it is possible to work on software development projects without in-depth knowledge of data structures and algorithms (DSA), mastering DSA will make you a much better programmer.
This expertise allows you to write optimized code that improves performance, which can save a company millions of dollars by avoiding problems associated with poorly written, inefficient code. Over time, mastering DSA leads to the development of robust, scalable, and high-performance applications.
The Key Benefits of Learning DSA
- Efficient Problem Solving:
- Why It’s Important: DSA gives you the tools to solve problems efficiently. If you know how to structure data and choose the right algorithm, you can approach problems methodically and implement solutions that are both effective and efficient.
- Example: When developing a function that requires searching or sorting large amounts of data (e.g., searching for users in a database), DSA knowledge will help you select the most appropriate algorithm (e.g., binary search or quicksort) that minimizes time and resource consumption.
- Optimized Code Performance:
- Why It’s Important: Performance is critical in web applications, as slow responses can lead to a poor user experience and even loss of users. Efficient data structures and algorithms ensure that your code runs faster and uses less memory.
- Example: If you are developing a recommendation system in an e-commerce application, using hash tables or balanced trees can speed up the recommendation process, even if you are working with millions of products.
- Scalability:
- Why It’s Important: As your web application grows, efficiently handling larger data sets and more users becomes critical. With DSA skills, you can design systems that scale easily without becoming bottlenecks.
- Example: If you’re designing the backend for a social media platform, understanding graph algorithms can help you implement efficient friend suggestion features or recognize communities within the network.
- Better Code Quality:
- Why This Is Important: Code that is both clear and efficient is easier to maintain, debug, and extend. DSA encourages you to think critically about how you structure your code, resulting in more modular, reusable, and cleaner implementations.
- Example: Using appropriate data structures such as queues or stacks can simplify complex logic in your code, making it easier to understand and change in the future.
- Effective Resource Management:
- Why It Matters: In web development, especially in full-stack roles, managing resources such as CPU, memory, and network bandwidth is critical. DSA skills allow you to optimize the use of these resources.
- Example: When handling file uploads or processing large data sets, using algorithms that minimize memory usage or reduce computational complexity can significantly improve performance and reduce costs.
- Dealing with Edge Cases and Large Inputs:
- Why It’s Important: Web applications often have to deal with unexpected edge cases or large inputs that can cause poorly designed algorithms to fail. DSA helps you anticipate these scenarios and handle them effectively.
- Example: Efficiently implementing pagination in a database query to process millions of records requires an understanding of algorithmic complexity and how to minimize load times.
- Fundamentals for Advanced Topics:
- Why It’s Important: Many advanced web development concepts, such as caching, load balancing, and search engine optimization (SEO), rely on basic DSA principles.
- Example: Implementing efficient caching mechanisms (e.g., LRU cache) requires an understanding of algorithms and data structures to ensure your application remains fast and responsive.
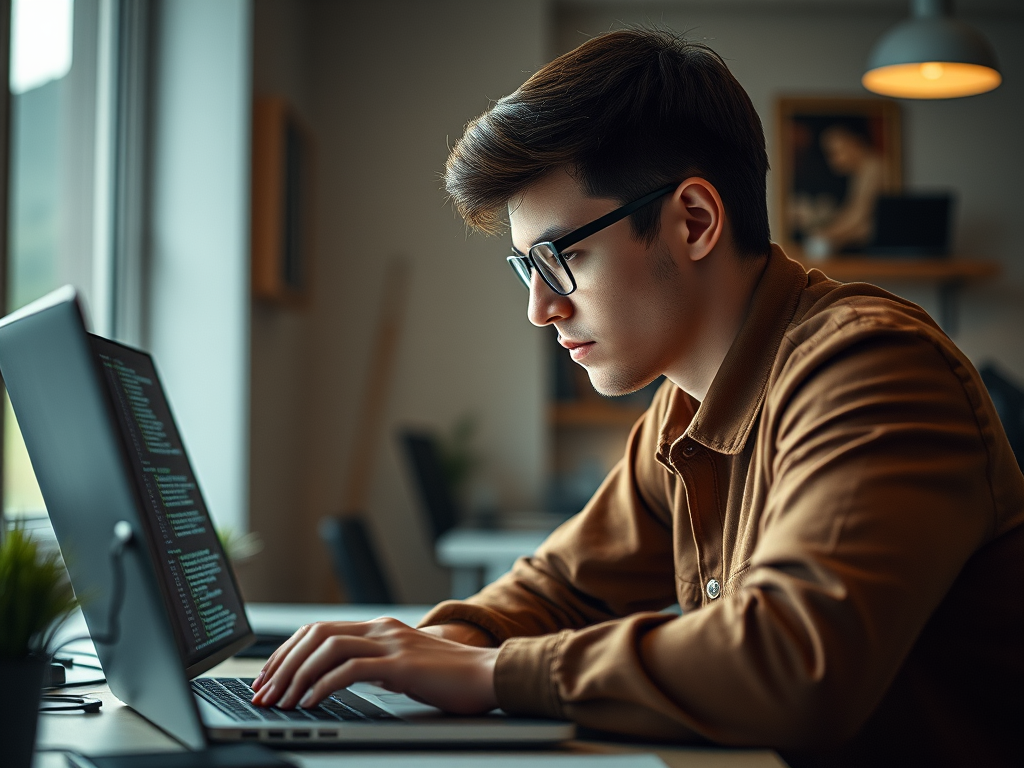
- Interviews and Professional Development:
- Why It’s Important: DSA skills are often a major topic in technical job interviews, especially for full-stack developers. Demonstrating your DSA skills can open up better job opportunities and advance your career.
- Example: Companies like Google, Facebook, and Amazon place a high value on DSA in their hiring processes, as they are looking for developers who can write optimized, high-quality code.
- Security:
- Why It’s Important: Security is a critical aspect of web development. If you understand DSA, you can write code that is less vulnerable to attacks such as SQL injection or buffer overflow.
- Example: Using proper data validation and sanitization techniques based on an understanding of data structures and processing can prevent common security vulnerabilities.
- Algorithmic Thinking:
- Why It’s Important: DSA encourages a mindset of algorithmic thinking, where you break problems down into smaller, more manageable pieces and approach them methodically. This approach is beneficial not only in programming, but also in software development as a whole.
- Example: If you are designing a new function or module, thinking in terms of algorithms and data structures will help you develop a more efficient and robust solution.
Conclusion:
Mastering data structures and algorithms is not just about passing technical interviews; it’s about equipping yourself with the knowledge and tools to write better, more efficient, and scalable code. For full-stack web developers, this understanding is essential to building powerful applications that meet real-world needs, scale effectively, and provide a seamless user experience.
What Are Search Algorithms in DSA?
Search algorithms in DSA are methods that are used to find a specific element within a list of elements, e.g., in arrays, linked lists, files, or databases. These algorithms are important to determine whether a particular element is present in a collection and, if so, to determine its index.
The Importance of Search Algorithms in DSA:
Search algorithms provide a more efficient way to find needed data in large data sets. Without a suitable search technique, finding an item in a dataset would be more time-consuming and less cost-efficient.
Practical Examples of Search Algorithms in DSA
- Searching for a Name or Number in a Large Data Set:
- Example: In a crime investigation, searching for a person’s name and phone number based on specific criteria in a large dataset requires efficient search algorithms.
- Searching for an Address in Google Maps:
- Example: This requires a highly complex search algorithm that includes techniques such as geocoding, indexing, search ranking, real-time data integration, and spatial search.
- Search Engine Queries:
- Example: Normal search queries in search engines such as Google, Bing, or Yahoo are also examples of advanced search algorithms.
Common Search Algorithms in DSA
There are various search algorithms in DSA, each with its own meaning and purpose.
Linear Search | O(n) |
Binary Search | O(nlogn) |
Jump Search | O(1) |
Interpolation Search | O(1) |
Exponential Search | O(1) |
Fibonacci Search | O(1) |
Ternary Search | O(nlogn) |
Depth-First Search (DFS) | O(V) |
Breadth-First Search (BFS) | O(V) |
Linear Search Algorithm
The most basic algorithm is the linear search algorithm.
If you master these search algorithms, you can significantly improve the efficiency of your code, especially when dealing with large data sets in real-world applications.
Linear Search Algorithm in DSA
Let’s delve into the Linear Search Algorithm—one of the most fundamental DSA search algorithms for locating the position (or presence) of an element in a given collection.
Understanding Linear Search
The Linear Search Algorithm starts by scanning the collection from the very first element, comparing each element with the target element you’re searching for.
If a match is found, it immediately returns the index of that element and the search process ends.
If no match is found, the algorithm continues this comparison process sequentially through the entire collection.
If the target element is not found by the time the algorithm reaches the last element, it returns a “not found” result.
Conversely, if the element is found somewhere within the collection, the algorithm returns the index of the element along with a “found” status.
This entire comparison process happens within a loop, making it straightforward but less efficient for large datasets compared to more advanced DSA search algorithms.
For linear Search to work the input collection doesn’t have to be in a sorted order. It can be either sorted or unsorted.
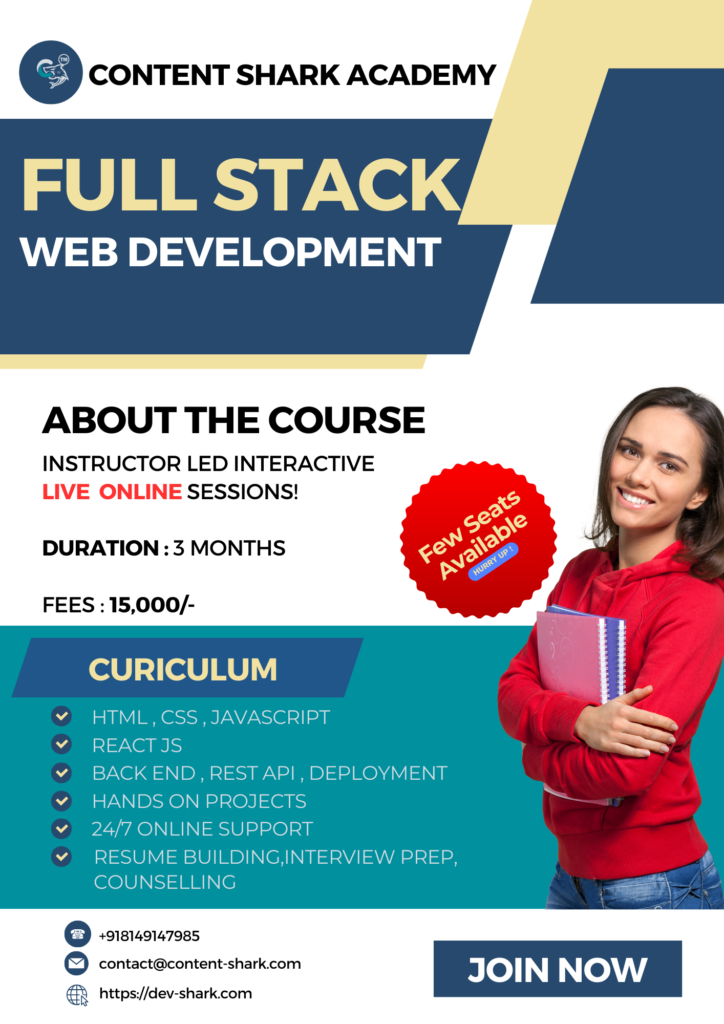
Example of Linear Search Algorithm in Action
Consider an array of elements:
let searchList = [11, 5, 17, 9];
Now, let’s say you want to search for element 9 within this list. The Linear Search Algorithm will initiate the comparison from the very first element in searchList.
Here’s how it works step by step:
- First comparison: Is 9 equal to 11?
- Result: No match, continue to the next element.
- Second comparison: Is 9 equal to 5?
- Result: No match, continue to the next element.
- Third comparison: Is 9 equal to 17?
- Result: No match, continue to the next element.
- Fourth comparison: Is 9 equal to 9?
- Result: Match found, return index 3 and terminate search.
In this example, the algorithm successfully finds element 9 at index
3. If the element wasn’t in the list, the algorithm would have continued until the last element and then returned a “not found” status.
Why Linear Search is Important in DSA
Although Linear Search is a basic method among DSA search algorithms, it is a critical foundation that helps understand more complex algorithms.
It’s especially useful for smaller datasets or unsorted collections where other, more advanced search algorithms may not be applicable.
Mastering Linear Search and other DSA search algorithms is crucial for optimizing your code and efficiently handling search operations in various real-world applications.
let prompt = require("prompt-sync")();
let num = +prompt("Enter number to search : ");
function LinearSearch(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] == target) {
return i;
}
}
return -1
}
var arr = [12, 65, 37, 43, 11]
var isPresent = LinearSearch(arr, num);
if(isPresent !== -1){
console.log("Element Found at Index Position: ", isPresent)
}
else {
console.log("element not present in the list")
}
Binary Search Algorithm in DSA
Binary Search Algorithms takes a completely different approach to find an element from a collection.
It is more efficient compared to Linear Search Algorithms when it comes to performance analysis. But for Binary Search to work the input collection must be in assorted order otherwise binary search will not work.
Binary Search follows the following steps.
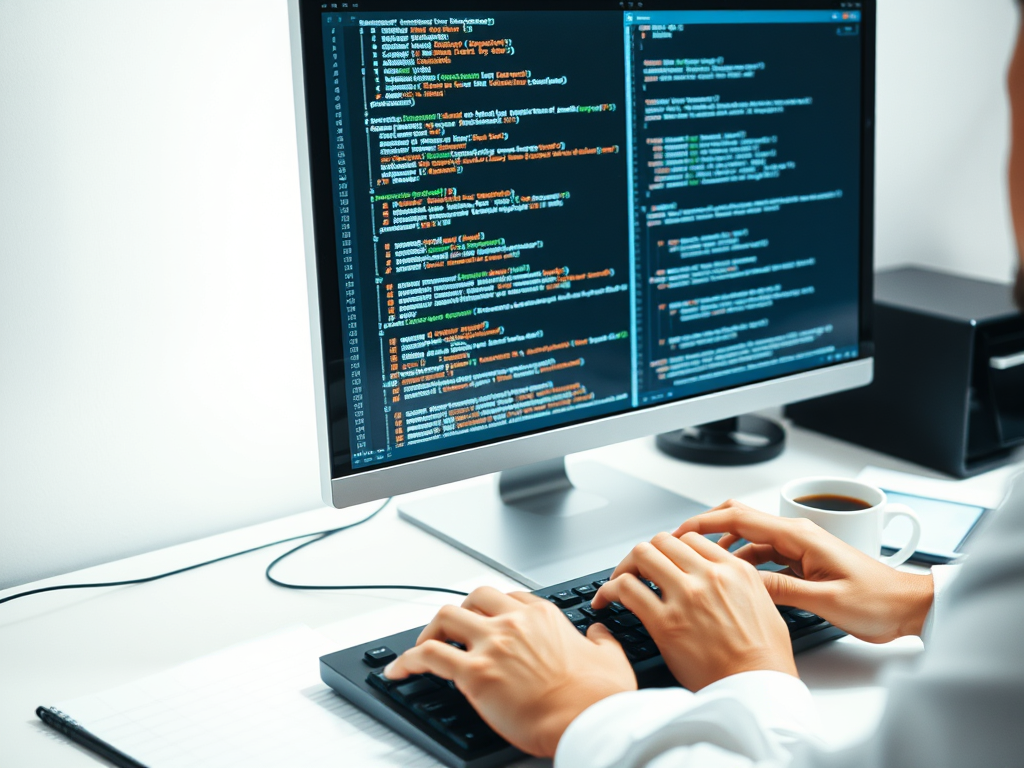
Let’s assume our input collection is in ascending order,
- In Binary Search Algorithm the input collection is first divided into three sub collections. If we are working with array then three sub-arrays
- Left sub-array
- middle Element
- right sub-array
let’s assume our input array is
arr1 = [ 2,7,17,32,67 ] and it is already sorted.
if it is not sorted then we need to first sort it before we try to apply binary search.
Now We have to find the middle element. So To find the middle element we need to figure out a way to find the index of the middle element.
The most common approach to find out the middle element is
indexMiddle = (firstIndex + lastIndex) / 2;
In this case
indexMiddle = (0 + 4) / 2 = 2;
So, the middle element is arr1[2] = 17.
Now, we have:
- Left sub-array: [2, 7]
- Middle element: 17
- Right sub-array: [32, 67]
- Comparison:
- If the target element is equal to the middle element, the search is successful, and the algorithm returns the index of the middle element (indexMiddle).
- If the target element is less than the middle element, the algorithm will recursively repeat the steps on the left sub-array.
- If the target element is greater than the middle element, the algorithm will recursively repeat the steps on the right sub-array.
In our example, since the target element 32 is greater than the middle element 17, the Binary Search will now focus on the right sub-array [32, 67].
- Repeating the Process: The algorithm will continue this process of dividing and comparing until it either finds the target element or determines that the element is not in the collection.
For the right sub-array [32, 67], the middle element is 32, which matches our target element. Therefore, the search is successful, and the algorithm returns the index where 32 is located in the original array.
Conclusion
The Binary Search Algorithm is highly efficient compared to linear search, especially for large datasets, due to its logarithmic time complexity. However, it requires the input collection to be sorted. By understanding and implementing Binary Search, you can significantly optimize search operations in your applications, making it an essential tool in the DSA search algorithms toolkit.
Binary Search Recursion
let prompt = require("prompt-sync")();
let target = +prompt("Enter number to search : ");
function RecursiveBinarySearch(arr, target, start, end) {
if (start > end) {
return;
}
let mid = Math.floor((start + end) / 2);
if (arr[mid] == target) {
return mid
}
if (arr[mid] > target) {
return RecursiveBinarySearch(arr, target, start, mid - 1)
}
else {
return RecursiveBinarySearch(arr, target, mid + 1, end)
}
}
var arr = [12, 65, 37, 43, 11];
arr = arr.sort();
var isPresent = RecursiveBinarySearch(arr, target, 0, arr.length - 1)
if( isPresent < 0){
console.log("Element Not Found")
}
else{
console.log("Element found at Index : ", isPresent)
}
CONCLUSION
In conclusion, both Linear Search and Binary Search play crucial roles in problem-solving and data retrieval. Linear Search is straightforward and works well for smaller datasets or unsorted arrays, but its time complexity (O(n)) makes it inefficient for larger data sets. On the other hand, Binary Search is highly efficient (O(log n)) but requires a sorted array to function. Understanding when to use each algorithm is key for optimizing performance. By mastering these algorithms, you not only enhance your coding efficiency but also prepare yourself for more advanced search techniques in computer science.